PROJECT: BrainTrain
This project portfolio page showcases my contributions to the BrainTrain project such as coding, documentation and other contributions.
Overview
All National University of Singapore(NUS) School of Computing(SoC) students have to take a software engineering module part encoded CS2103T as a required module. Our team comprises of 5 members who were tasked to develop and enhance an existing address book application within 7 weeks.
We were given a choice to morph the existing address book to any application we want and we chose to develop BrainTrain, which will be detailed below.
BrainTrain uses Spaced-Repetition System (SRS), a more effective and efficient memorisation technique compared to hardcore memorisation techniques. Users will be able to learn more with less time. User interaction happens through Command Line Interface (CLI) and it has a minimal Graphical User Interface (GUI) created with JavaFX. It is written in Java, and has about 10 kLoC.
My role in BrainTrain is to design and manage the user data through the manipulation of importing and exporting of files. The following sections give a summary of how I have contributed to the project and showcases my ability to write both the user and developer guide.
Summary of contributions
-
Major enhancement: User features, Comma-Separated Values(CSV) File Storage
-
What it does: User features manage the user progress and import and export their progress into a simple CSV file accordingly. This feature is automated.
Import of user file happens when the application is opened.
Export of user file happens when the application is closed. -
Why it is important: In consideration of the portability for users to use BrainTrain across different devices, it is important to have a file to keep track of the status of the questions. Furthermore, each question will have a unique SRS-date that is dependent on how well the user answered the questions. Questions with approaching due dates will be listed first.
-
-
Minor enhancement: Sanitise user inputs of the CSV file that is generated
-
Code contributed: [RepoSense]
-
Other contributions:
Contributions to the User Guide
Start of extract of user mode
User Transfer mode
You can use BrainTrain across different devices. This is an in-built feature that is automated in BrainTrain.
Using BrainTrain across different devices
To transfer your files:
-
Store the
userdata
andlessons
folder into your transfer device (thumbdrive etc.). -
On another device, ensure that BrainTrain has been executed
at least once
. -
Transfer and overwrite the
userdata
andlessons
folder. -
Your progress will be automatically imported on that device.
Future enhancements
Importing data from website [Coming in v2.0]
Easily transfer files using import command to get files directly from a specific link without the use of transfer devices.
The usage of hard drives to transfer and pull documents has reduced overtime as new cloud storage are introduced. Thus, with this new feature, it will help to pull the user file and lessons from links.
Example : import User l/[INSERT_DESIRED_LINK_HERE]
End of extract of user mode
Click to read the full User Guide.
Contributions to the Developer Guide
Start of extract of data storage
User storage feature
The user storage features implements the following functions:
-
Parsing user data into the correct format for file saving
-
Parsing file into the correct format for user data
These functions are automatically performed on opening/closing the application. File I/O is handled in the CSVUtil class.
|
User format

The user is parsed by CsvUserStorage
, which is converted between User and List<String[]> format. List<String[]> format is the primary format handled by CsvUtil
for reading and writing to .csv
files.
A few noteworthy mentions of the main components saved into a .csv
file for UserStorage are listed below.
Hashcode
This is the question hashcode. Every question will have a unique hashcode for identification.
SRSDueDate
This is the srsDueDate generated for that question. Questions with a nearer due-date will be generated first in the quiz.
Difficult
These are questions that are labelled difficult by the user. Users are able to specifically practise questions that they have labelled difficult in session.
Sequence diagram for user storage
Below is a sequence diagram of how a file is parsed into BrainTrain
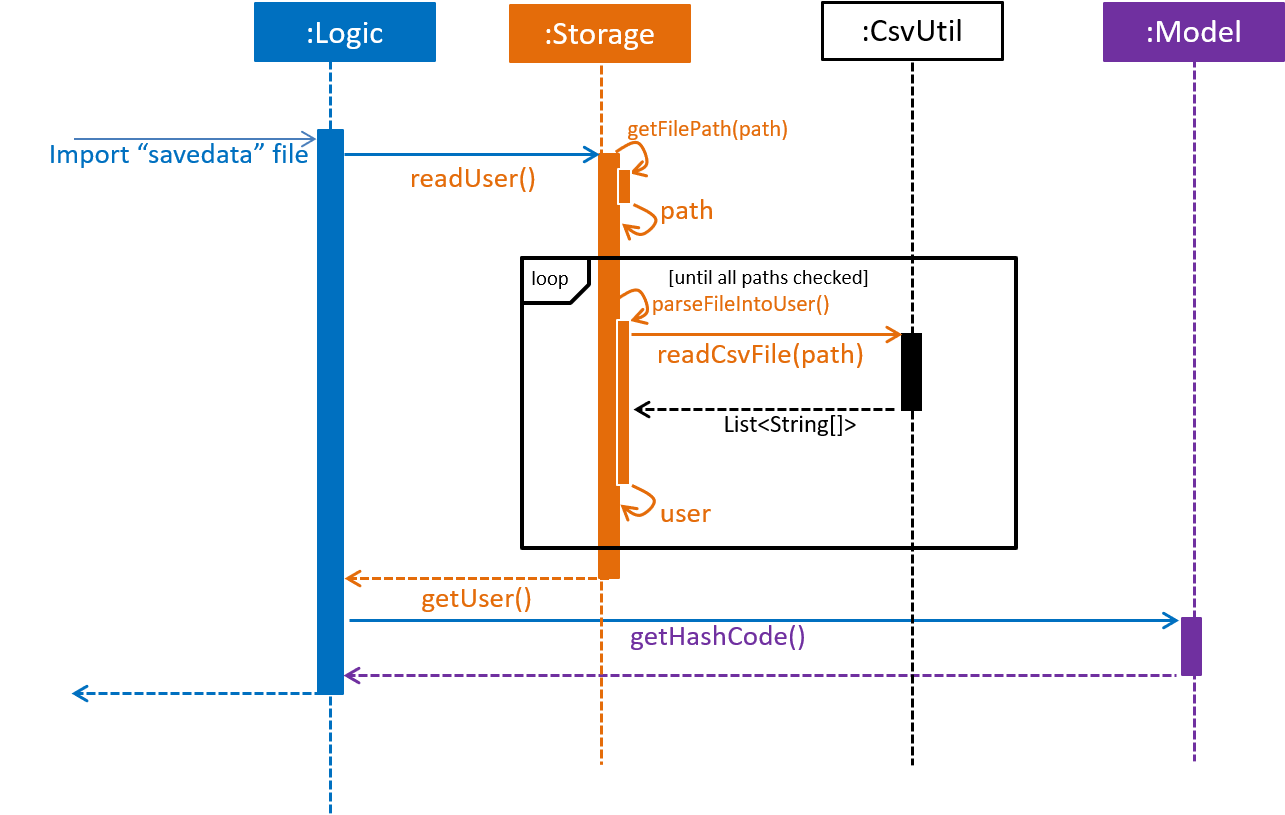
Code snippet
The following code snippet shows how the user data is obtained:
private Optional<CardSrsData> parseStringIntoCard(String[] cardArray) throws
NumberFormatException, DateTimeParseException {
for (int i = 0; i < cardArray.length - 1; i++) {
if (cardArray[i].isEmpty()) {
logger.warning("There are empty values in the file");
return Optional.empty();
}
}
try {
hashCode = Integer.parseInt(cardArray[0]);
numOfAttempts = Integer.parseInt(cardArray[1]);
streak = Integer.parseInt(cardArray[2]);
srs = Instant.parse(cardArray[3]);
isDifficult = cardArray[4].equals("true");
if (hashCode == ZERO) {
logger.warning("Hashcode cannot be 0 in " + filePath.toString());
return Optional.empty();
}
} catch (NumberFormatException e) {
logger.warning("Values are not correct in " + filePath.toString());
return Optional.empty();
}
card = new CardSrsData(hashCode, numOfAttempts, streak, srs, isDifficult);
return Optional.of(card);
}
Design considerations
-
Alternative 1 (Current choice): Automate importing/exporting when session is open/close
-
Pros: Lesser commands to manage.
-
Cons: Opening and closing may take up a lot of time if file is very big.
-
-
Alternative 2: Individual commands for user to toggle with to import and export files when desired
-
Pros: More control given to user
-
Cons: Accidental overwriting of files may occur. Furthermore, if users are not able to parse in values correctly, the file will not be saved. This is troublesome for users who are not tech-savvy.
-
Saving user data
-
Ensuring that there is a save user file generated. The file should be generated under
/userdata/savedata
-
Prerequisites: The user has at least started on a quiz once.
-
NOTE: There will be 5 unique notations generated in the
savedata
file. It is arranged in an array as such [hashcode, number of attempts, streak, SRS date, isDifficult] -
Test case: Open the
savedata
file and modifyhashcode = 0
Expected: Launch braintrain.jar again and open braintrain.log. There should be a warning message stating:"WARNING: There are empty values in the file"
Other incorrect cases: Settingnumber of attempts < 0
orstreak < 0
.
-
End of extract of data storage
Click to read the full Developer Guide.